Creating the admin catalog content
In this lesson we will create the table to display the orders to the admin user that will allow that person to view the product catalog in tabular form. Open the admin-catalog.component.ts and add the following code:
1import { Component, inject } from '@angular/core';
2import { PageEvent } from '@angular/material/paginator';
3import { ShopService } from '../../../core/services/shop.service';
4import { Product } from '../../../shared/models/product';
5import { ShopParams } from '../../../shared/models/shopParams';
6import { CustomTableComponent } from '../../../shared/components/custom-table/custom-table.component';
7import { MatButtonModule } from '@angular/material/button';
8
9@Component({
10 selector: 'app-admin-catalog',
11 standalone: true,
12 imports: [
13 CustomTableComponent,
14 MatButtonModule
15 ],
16 templateUrl: './admin-catalog.component.html',
17 styleUrl: './admin-catalog.component.scss'
18})
19export class AdminCatalogComponent {
20 products: Product[] = [];
21 private shopService = inject(ShopService);
22 productParams = new ShopParams();
23 totalItems = 0;
24
25 columns = [
26 { field: 'id', header: 'No.' },
27 { field: 'name', header: 'Product name' },
28 { field: 'type', header: 'Type' },
29 { field: 'brand', header: 'Brand' },
30 { field: 'quantityInStock', header: 'Quantity' },
31 { field: 'price', header: 'Price', pipe: 'currency', pipeArgs: 'USD' }
32 ];
33
34 actions = [
35 {
36 label: 'Edit',
37 icon: 'edit',
38 tooltip: 'Edit product',
39 action: (row: any) => {
40 console.log(row)
41 }
42 },
43 {
44 label: 'Delete',
45 icon: 'delete',
46 tooltip: 'Delete product',
47 action: (row: any) => {
48 console.log(row)
49 }
50 },
51 {
52 label: 'Update quantity',
53 icon: 'add_circle',
54 tooltip: 'Update quantity in stock',
55 action: (row: any) => {
56 console.log(row)
57 }
58 },
59 ];
60
61 ngOnInit(): void {
62 this.loadProducts();
63 }
64
65 loadProducts() {
66 this.shopService.getProducts(this.productParams).subscribe({
67 next: response => {
68 if (response.data) {
69 this.products = response.data;
70 this.totalItems = response.count;
71 }
72 }
73 })
74 }
75
76 onPageChange(event: PageEvent) {
77 this.productParams.pageNumber = event.pageIndex + 1;
78 this.productParams.pageSize = event.pageSize;
79 this.loadProducts();
80 }
81}
82
In this component we are simply using the existing shop service to get a paginated list of products and are creating the columns and actions array we need to pass to the custom table.
Open the template for this component and add the following code:
1<div class="flex justify-between items-center mt-2">
2 <div class="text-2xl font-semibold mt-4">
3 Admin catalog
4 </div>
5 <button mat-flat-button>
6 Create new
7 </button>
8</div>
9
10<app-custom-table
11 [columns]="columns"
12 [dataSource]="products"
13 [actions]="actions"
14 [totalItems]="totalItems"
15 [pageSize]="productParams.pageSize"
16 (pageChange)="onPageChange($event)"
17/>
Once again, we are using the custom-table to create our table and passing the columns and actions to this, as well as the list of products and pagination data.
Update the admin.component.html and add this new component to the template (also add the import for this into the component):
1<div class="min-h-screen">
2 <mat-tab-group class="bg-white">
3 <mat-tab label="Orders">
4 <app-admin-orders />
5 </mat-tab>
6 <mat-tab label="Catalog">
7 <app-admin-catalog />
8 </mat-tab>
9 <mat-tab label="Customer service">
10 Customer service
11 </mat-tab>
12 </mat-tab-group>
13</div>
14
If you browse to the admin page catalog tab you should now have the following:
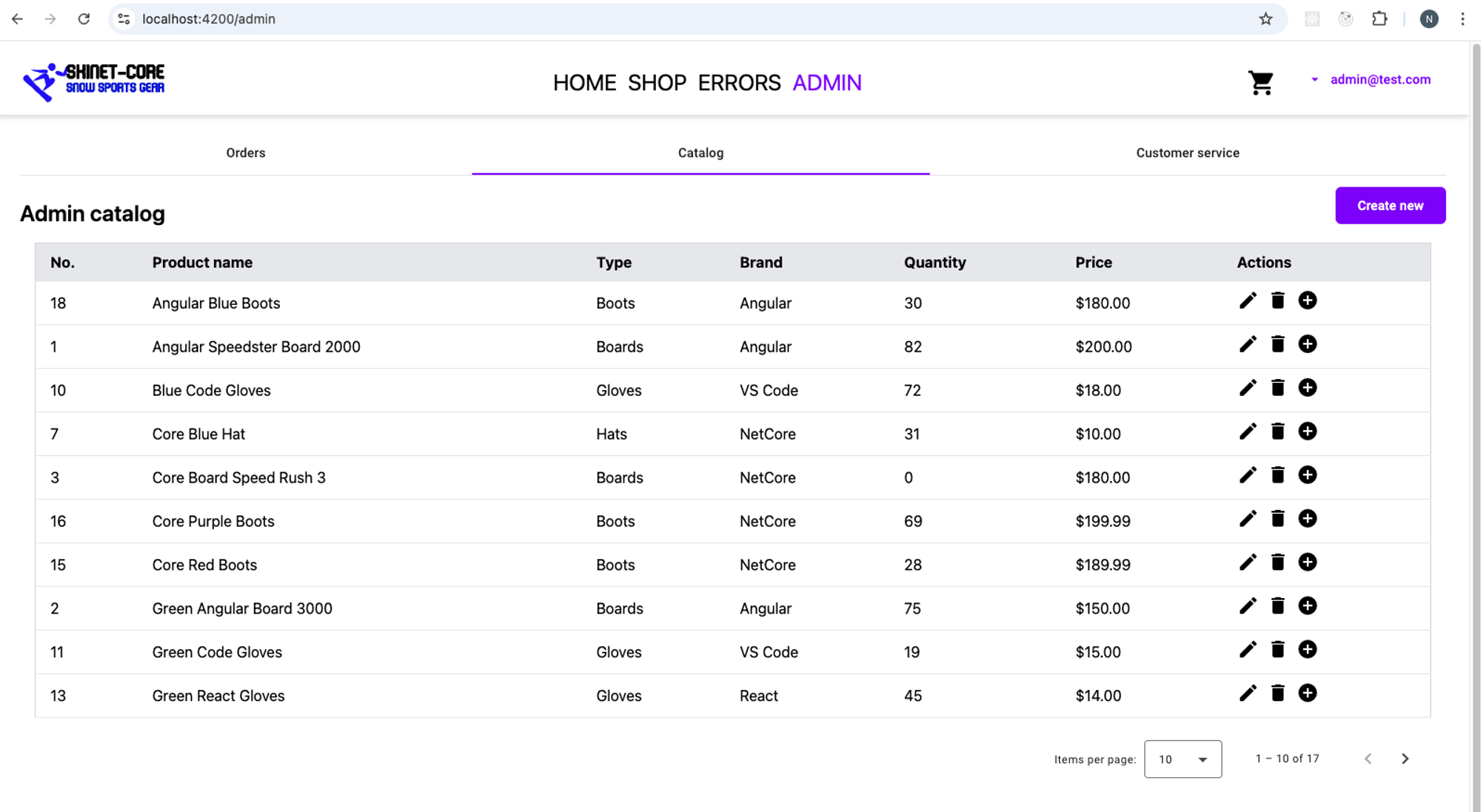
If you click on any of the buttons you should see the output in the console of chrome dev tools.
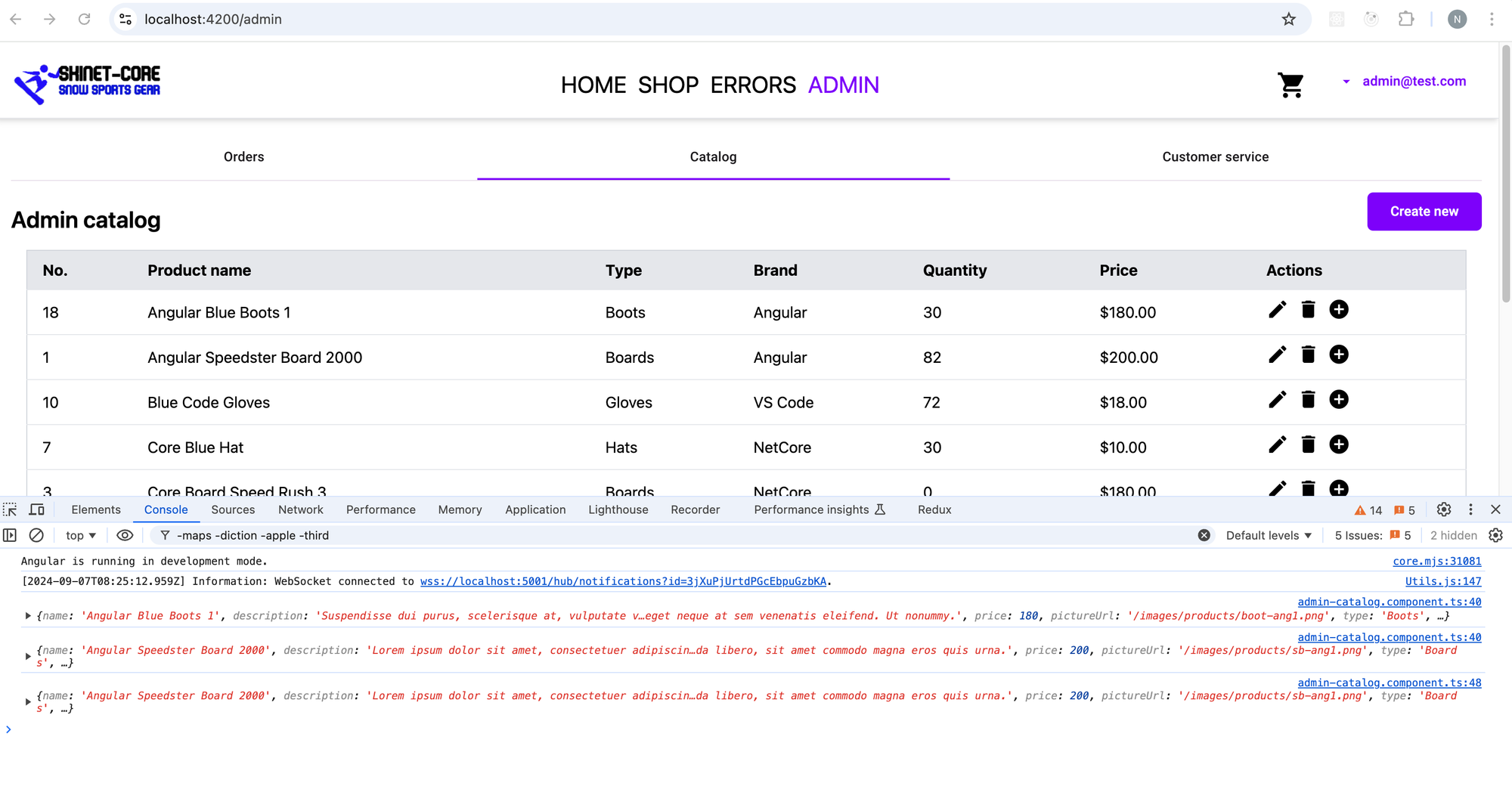
That’s it for this lesson. In the next we will look at creating the product form.